Improving icons visibility
Our navbar gained a nice scroll animation, but the icons on the right side lost their visibility.
We need to improve this.

Step 1 - add .text-white
to the icons
In each <a>
element inside
<div id="navbar-icons">
we need to replace the
..text-neutral-600
class with the
.text-white
class:
<!-- Right elements -->
<div id="navbar-icons" class="relative flex items-center">
<!-- YouTube Icon -->
<a
class="me-4 text-white transition duration-200 hover:text-neutral-700 hover:ease-in-out focus:text-neutral-700 disabled:text-black/30 motion-reduce:transition-none dark:text-neutral-200 dark:hover:text-neutral-300 dark:focus:text-neutral-300 [&.active]:text-black/90 dark:[&.active]:text-neutral-400"
href="#">
[...]
</a>
[...]
</div>
Step 2 - update animated navbar javascript
We need to make a small update to our src/js/index.js
file, so
it includes the icons:
// Initialization for ES Users
import { Carousel, initTWE } from 'tw-elements';
initTWE({ Carousel });
// Animated navbar
// We store the navbar element in a variable using the getElementById method and the id of the navbar
const animatedNavbar = document.getElementById('animated-navbar');
// We store the navbar-icons 'div' in a variable using the getElementById method and the id of the 'div' containing icons
const navbarIcons = document.getElementById('navbar-icons');
// We store the 'a' elements in a variable using the querySelectorAll method and the 'a' elements inside the navbar-icons 'div'
const navIconLinks = navbarIcons.querySelectorAll('a');
// If windows.scrollY > 0 (that means the user has scrolled down) we add an event listener
window.addEventListener('scroll', function () {
if (window.scrollY > 0) {
// We add the .bg-white class to the navbar when user scrolls down
animatedNavbar.classList.add('bg-white');
// We remove the .text-white class from the 'a' elements inside the navbar-icons 'div' and add the .text-neutral-500 class
navIconLinks.forEach((link) => {
link.classList.remove('text-white');
link.classList.add('text-neutral-500');
});
} else {
// We remove the .bg-white class from the navbar when user scrolls up to the top of the page
animatedNavbar.classList.remove('bg-white');
// We add the .text-white class to the 'a' elements inside the navbar-icons 'div' and remove the .text-neutral-500 class when user scrolls up to the top of the page
navIconLinks.forEach((link) => {
link.classList.add('text-white');
link.classList.remove('text-neutral-500');
});
}
});
What we do here:
-
We store the
<div id="navbar-icons">
with its content in a variable using thegetElementById
method and the id of the<div>
containing icons -
We store the
<a>
elements in a variable using thequerySelectorAll
method and the<a>
elements inside the<div id="navbar-icons">
-
We remove the
.text-white
class from the<a>
elements inside the<div id="navbar-icons">
and add the.text-neutral-500
class -
We add the
.text-white
class to the<a>
elements inside the<div id="navbar-icons">
and remove the.text-neutral-500
class when user scrolls up to the top of the page
Now the icons are white when the navbar is transparent and in the initial position, and they take on a more delicate gray color when we start scrolling and the navbar becomes white.

Step 3 - change the height on scroll
We can further diversify our scroll animation by changing the height of the navbar (specifically by changing its padding).
Update src/js/index.js
as follow:
// Initialization for ES Users
import { Carousel, initTWE } from 'tw-elements';
initTWE({ Carousel });
// Animated navbar
// We store the navbar element in a variable using the getElementById method and the id of the navbar
const animatedNavbar = document.getElementById('animated-navbar');
// We store the navbar-icons 'div' in a variable using the getElementById method and the id of the 'div' containing icons
const navbarIcons = document.getElementById('navbar-icons');
// We store the 'a' elements in a variable using the querySelectorAll method and the 'a' elements inside the navbar-icons 'div'
const navIconLinks = navbarIcons.querySelectorAll('a');
// If windows.scrollY > 0 (that means the user has scrolled down) we add an event listener
window.addEventListener('scroll', function () {
if (window.scrollY > 0) {
// We add the .bg-white class to the navbar when user scrolls down
animatedNavbar.classList.add('bg-white');
// We remove the .lg:py-4 class and add the .lg:py-5 class to the navbar when user scrolls down
animatedNavbar.classList.remove("lg:py-4");
animatedNavbar.classList.add("lg:py-5");
// We remove the .text-white class from the 'a' elements inside the navbar-icons 'div' and add the .text-neutral-500 class
navIconLinks.forEach((link) => {
link.classList.remove('text-white');
link.classList.add('text-neutral-500');
});
} else {
// We remove the .bg-white class from the navbar when user scrolls up to the top of the page
animatedNavbar.classList.remove('bg-white');
// We remove the .lg:py-5 class and add the .lg:py-4 class to the navbar when user scrolls up to the top of the page
animatedNavbar.classList.remove("lg:py-5");
animatedNavbar.classList.add("lg:py-4");
// We add the .text-white class to the 'a' elements inside the navbar-icons 'div' and remove the .text-neutral-500 class when user scrolls up to the top of the page
navIconLinks.forEach((link) => {
link.classList.add('text-white');
link.classList.remove('text-neutral-500');
});
}
});
What we do here:
-
We remove the
.lg:py-4
class and add the.lg:py-5
class to the navbar when user scrolls down -
We remove the
.lg:py-5
class and add the.lg:py-4
class to the navbar when user scrolls up to the top of the page
To make the animation smooth, we also need to update our CSS code and add
padding 0.5s ease-in-out
to it:
<style>
#animated-navbar {
transition: background 0.5s ease-in-out, padding 0.5s ease-in-out;
}
</style>
Now look how nice and smooth our animation is:

So at the end this is how our index.html
file looks like:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta
name="viewport"
content="width=device-width, initial-scale=1, shrink-to-fit=no" />
<meta http-equiv="x-ua-compatible" content="ie=edge" />
<title>TE Vite Starter</title>
<!-- Favicon -->
<link
rel="icon"
href="https://tecdn.b-cdn.net/img/Marketing/general/logo/small/te.ico"
type="image/x-icon" />
<!-- Roboto font -->
<link
href="https://fonts.googleapis.com/css?family=Roboto:300,400,500,700,900&display=swap"
rel="stylesheet" />
<!-- Main css file -->
<link rel="stylesheet" href="src/scss/index.scss" />
<!-- Custom styles -->
<style>
#animated-navbar {
transition: background 0.5s ease-in-out, padding 0.5s ease-in-out;
}
</style>
</head>
<body>
<!--Main Navigation-->
<header>
<!-- Navbar -->
<nav
id="animated-navbar"
class="flex-no-wrap fixed top-0 z-10 flex w-full items-center justify-between py-2 dark:bg-neutral-600 dark:shadow-black/10 lg:flex-wrap lg:justify-start lg:py-4">
<div
class="flex w-full flex-wrap items-center justify-between px-3">
<!-- Hamburger button for mobile view -->
<button
class="block border-0 bg-transparent px-2 text-neutral-500 hover:no-underline hover:shadow-none focus:no-underline focus:shadow-none focus:outline-none focus:ring-0 dark:text-neutral-200 lg:hidden"
type="button"
data-twe-collapse-init
data-twe-target="#navbarSupportedContent1"
aria-controls="navbarSupportedContent1"
aria-expanded="false"
aria-label="Toggle navigation">
<!-- Hamburger icon -->
<span class="[&>svg]:w-7">
<svg
xmlns="http://www.w3.org/2000/svg"
viewBox="0 0 24 24"
fill="currentColor"
class="h-7 w-7">
<path
fill-rule="evenodd"
d="M3 6.75A.75.75 0 013.75 6h16.5a.75.75 0 010 1.5H3.75A.75.75 0 013 6.75zM3 12a.75.75 0 01.75-.75h16.5a.75.75 0 010 1.5H3.75A.75.75 0 013 12zm0 5.25a.75.75 0 01.75-.75h16.5a.75.75 0 010 1.5H3.75a.75.75 0 01-.75-.75z"
clip-rule="evenodd" />
</svg>
</span>
</button>
<!-- Collapsible navigation container -->
<div
class="!visible hidden flex-grow basis-[100%] items-center lg:!flex lg:basis-auto"
id="navbarSupportedContent1"
data-twe-collapse-item>
<!-- Logo -->
<a
class="mb-4 me-5 ms-2 mt-3 flex items-center text-neutral-900 hover:text-neutral-900 focus:text-neutral-900 dark:text-neutral-200 dark:hover:text-neutral-400 dark:focus:text-neutral-400 lg:mb-0 lg:mt-0"
href="#">
<img
src="./logo.png"
style="height: 20px"
alt="TE Logo"
loading="lazy" />
</a>
<!-- Left navigation links -->
<ul
class="list-style-none me-auto flex flex-col ps-0 lg:flex-row"
data-twe-navbar-nav-ref>
<li class="mb-4 lg:mb-0 lg:pe-2" data-twe-nav-item-ref>
<!-- Projects link -->
<a
class="text-neutral-500 transition duration-200 hover:text-neutral-700 hover:ease-in-out focus:text-neutral-700 disabled:text-black/30 motion-reduce:transition-none dark:text-neutral-200 dark:hover:text-neutral-300 dark:focus:text-neutral-300 lg:px-2 [&.active]:text-black/90 dark:[&.active]:text-zinc-400"
href="#"
data-twe-nav-link-ref
>Projects</a
>
</li>
<!-- About me link -->
<li class="mb-4 lg:mb-0 lg:pe-2" data-twe-nav-item-ref>
<a
class="text-neutral-500 transition duration-200 hover:text-neutral-700 hover:ease-in-out focus:text-neutral-700 disabled:text-black/30 motion-reduce:transition-none dark:text-neutral-200 dark:hover:text-neutral-300 dark:focus:text-neutral-300 lg:px-2 [&.active]:text-black/90 dark:[&.active]:text-neutral-400"
href="#"
data-twe-nav-link-ref
>About me</a
>
</li>
<!-- Testimonials link -->
<li class="mb-4 lg:mb-0 lg:pe-2" data-twe-nav-item-ref>
<a
class="text-neutral-500 transition duration-200 hover:text-neutral-700 hover:ease-in-out focus:text-neutral-700 disabled:text-black/30 motion-reduce:transition-none dark:text-neutral-200 dark:hover:text-neutral-300 dark:focus:text-neutral-300 lg:px-2 [&.active]:text-black/90 dark:[&.active]:text-neutral-400"
href="#"
data-twe-nav-link-ref
>Testimonials</a
>
</li>
<!-- Contact link -->
<li class="mb-4 lg:mb-0 lg:pe-2" data-twe-nav-item-ref>
<a
class="text-neutral-500 transition duration-200 hover:text-neutral-700 hover:ease-in-out focus:text-neutral-700 disabled:text-black/30 motion-reduce:transition-none dark:text-neutral-200 dark:hover:text-neutral-300 dark:focus:text-neutral-300 lg:px-2 [&.active]:text-black/90 dark:[&.active]:text-neutral-400"
href="#"
data-twe-nav-link-ref
>Contact</a
>
</li>
</ul>
</div>
<!-- Right elements -->
<div id="navbar-icons" class="relative flex items-center">
<!-- YouTube Icon -->
<a
class="me-4 text-white transition duration-200 hover:text-neutral-700 hover:ease-in-out focus:text-neutral-700 disabled:text-black/30 motion-reduce:transition-none dark:text-neutral-200 dark:hover:text-neutral-300 dark:focus:text-neutral-300 [&.active]:text-black/90 dark:[&.active]:text-neutral-400"
href="#">
<span class="[&>svg]:w-5">
<svg
xmlns="http://www.w3.org/2000/svg"
class="h-4 w-4"
viewBox="0 0 448 512"
fill="currentColor">
<!--! Font Awesome Free 6.4.2 by @fontawesome - https://fontawesome.com License - https://fontawesome.com/license (Commercial License) Copyright 2023 Fonticons, Inc. -->
<path
d="M186.8 202.1l95.2 54.1-95.2 54.1V202.1zM448 80v352c0 26.5-21.5 48-48 48H48c-26.5 0-48-21.5-48-48V80c0-26.5 21.5-48 48-48h352c26.5 0 48 21.5 48 48zm-42 176.3s0-59.6-7.6-88.2c-4.2-15.8-16.5-28.2-32.2-32.4C337.9 128 224 128 224 128s-113.9 0-142.2 7.7c-15.7 4.2-28 16.6-32.2 32.4-7.6 28.5-7.6 88.2-7.6 88.2s0 59.6 7.6 88.2c4.2 15.8 16.5 27.7 32.2 31.9C110.1 384 224 384 224 384s113.9 0 142.2-7.7c15.7-4.2 28-16.1 32.2-31.9 7.6-28.5 7.6-88.1 7.6-88.1z" />
</svg>
</span>
</a>
<!-- Facebook Icon -->
<a
class="me-4 text-white transition duration-200 hover:text-neutral-700 hover:ease-in-out focus:text-neutral-700 disabled:text-black/30 motion-reduce:transition-none dark:text-neutral-200 dark:hover:text-neutral-300 dark:focus:text-neutral-300 [&.active]:text-black/90 dark:[&.active]:text-neutral-400"
href="#">
<span class="[&>svg]:w-5">
<svg
xmlns="http://www.w3.org/2000/svg"
class="h-4 w-4"
viewBox="0 0 512 512"
fill="currentColor">
<!--! Font Awesome Free 6.4.2 by @fontawesome - https://fontawesome.com License - https://fontawesome.com/license (Commercial License) Copyright 2023 Fonticons, Inc. -->
<path
d="M504 256C504 119 393 8 256 8S8 119 8 256c0 123.78 90.69 226.38 209.25 245V327.69h-63V256h63v-54.64c0-62.15 37-96.48 93.67-96.48 27.14 0 55.52 4.84 55.52 4.84v61h-31.28c-30.8 0-40.41 19.12-40.41 38.73V256h68.78l-11 71.69h-57.78V501C413.31 482.38 504 379.78 504 256z" />
</svg>
</span>
</a>
<!-- X Twitter Icon -->
<a
class="me-4 text-white transition duration-200 hover:text-neutral-700 hover:ease-in-out focus:text-neutral-700 disabled:text-black/30 motion-reduce:transition-none dark:text-neutral-200 dark:hover:text-neutral-300 dark:focus:text-neutral-300 [&.active]:text-black/90 dark:[&.active]:text-neutral-400"
href="#">
<span class="[&>svg]:w-5">
<svg
xmlns="http://www.w3.org/2000/svg"
class="h-4 w-4"
viewBox="0 0 512 512"
fill="currentColor">
<!--! Font Awesome Free 6.4.2 by @fontawesome - https://fontawesome.com License - https://fontawesome.com/license (Commercial License) Copyright 2023 Fonticons, Inc. -->
<path
d="M389.2 48h70.6L305.6 224.2 487 464H345L233.7 318.6 106.5 464H35.8L200.7 275.5 26.8 48H172.4L272.9 180.9 389.2 48zM364.4 421.8h39.1L151.1 88h-42L364.4 421.8z" />
</svg>
</span>
</a>
<!-- Instagram Icon -->
<a
class="me-4 text-white transition duration-200 hover:text-neutral-700 hover:ease-in-out focus:text-neutral-700 disabled:text-black/30 motion-reduce:transition-none dark:text-neutral-200 dark:hover:text-neutral-300 dark:focus:text-neutral-300 [&.active]:text-black/90 dark:[&.active]:text-neutral-400"
href="#">
<span class="[&>svg]:w-5">
<svg
xmlns="http://www.w3.org/2000/svg"
class="h-4 w-4"
viewBox="0 0 448 512"
fill="currentColor">
<!--! Font Awesome Free 6.4.2 by @fontawesome - https://fontawesome.com License - https://fontawesome.com/license (Commercial License) Copyright 2023 Fonticons, Inc. -->
<path
d="M224,202.66A53.34,53.34,0,1,0,277.36,256,53.38,53.38,0,0,0,224,202.66Zm124.71-41a54,54,0,0,0-30.41-30.41c-21-8.29-71-6.43-94.3-6.43s-73.25-1.93-94.31,6.43a54,54,0,0,0-30.41,30.41c-8.28,21-6.43,71.05-6.43,94.33S91,329.26,99.32,350.33a54,54,0,0,0,30.41,30.41c21,8.29,71,6.43,94.31,6.43s73.24,1.93,94.3-6.43a54,54,0,0,0,30.41-30.41c8.35-21,6.43-71.05,6.43-94.33S357.1,182.74,348.75,161.67ZM224,338a82,82,0,1,1,82-82A81.9,81.9,0,0,1,224,338Zm85.38-148.3a19.14,19.14,0,1,1,19.13-19.14A19.1,19.1,0,0,1,309.42,189.74ZM400,32H48A48,48,0,0,0,0,80V432a48,48,0,0,0,48,48H400a48,48,0,0,0,48-48V80A48,48,0,0,0,400,32ZM382.88,322c-1.29,25.63-7.14,48.34-25.85,67s-41.4,24.63-67,25.85c-26.41,1.49-105.59,1.49-132,0-25.63-1.29-48.26-7.15-67-25.85s-24.63-41.42-25.85-67c-1.49-26.42-1.49-105.61,0-132,1.29-25.63,7.07-48.34,25.85-67s41.47-24.56,67-25.78c26.41-1.49,105.59-1.49,132,0,25.63,1.29,48.33,7.15,67,25.85s24.63,41.42,25.85,67.05C384.37,216.44,384.37,295.56,382.88,322Z" />
</svg>
</span>
</a>
</div>
</div>
</nav>
<!-- Navbar -->
<!-- Section: Split screen -->
<section class="">
<!-- Grid -->
<div class="grid h-screen grid-cols-1 lg:grid-cols-2">
<!-- First column -->
<div class="hidden lg:block">
<!-- Outer wrapper for flexbox -->
<div class="flex h-full flex-col items-center justify-center">
<!-- Inner flexbox wrapper for headings -->
<div class="flex h-full items-center">
<!-- Headings -->
<div class="text-gray-600">
<h1 class="mb-5 text-6xl font-light">John Doe</h1>
<h2 class="mb-7 text-6xl font-bold uppercase">
Web developer
</h2>
</div>
</div>
<!-- Inner flexbox wrapper for CTA elements -->
<div
class="flex w-full items-center justify-between px-10 pb-8">
<a href="" target="_blank">
<svg
xmlns="http://www.w3.org/2000/svg"
class="h-6 w-6"
viewBox="0 0 496 512">
<!--! Font Awesome Free 6.4.2 by @fontawesome - https://fontawesome.com License - https://fontawesome.com/license (Commercial License) Copyright 2023 Fonticons, Inc. -->
<path
d="M165.9 397.4c0 2-2.3 3.6-5.2 3.6-3.3.3-5.6-1.3-5.6-3.6 0-2 2.3-3.6 5.2-3.6 3-.3 5.6 1.3 5.6 3.6zm-31.1-4.5c-.7 2 1.3 4.3 4.3 4.9 2.6 1 5.6 0 6.2-2s-1.3-4.3-4.3-5.2c-2.6-.7-5.5.3-6.2 2.3zm44.2-1.7c-2.9.7-4.9 2.6-4.6 4.9.3 2 2.9 3.3 5.9 2.6 2.9-.7 4.9-2.6 4.6-4.6-.3-1.9-3-3.2-5.9-2.9zM244.8 8C106.1 8 0 113.3 0 252c0 110.9 69.8 205.8 169.5 239.2 12.8 2.3 17.3-5.6 17.3-12.1 0-6.2-.3-40.4-.3-61.4 0 0-70 15-84.7-29.8 0 0-11.4-29.1-27.8-36.6 0 0-22.9-15.7 1.6-15.4 0 0 24.9 2 38.6 25.8 21.9 38.6 58.6 27.5 72.9 20.9 2.3-16 8.8-27.1 16-33.7-55.9-6.2-112.3-14.3-112.3-110.5 0-27.5 7.6-41.3 23.6-58.9-2.6-6.5-11.1-33.3 2.6-67.9 20.9-6.5 69 27 69 27 20-5.6 41.5-8.5 62.8-8.5s42.8 2.9 62.8 8.5c0 0 48.1-33.6 69-27 13.7 34.7 5.2 61.4 2.6 67.9 16 17.7 25.8 31.5 25.8 58.9 0 96.5-58.9 104.2-114.8 110.5 9.2 7.9 17 22.9 17 46.4 0 33.7-.3 75.4-.3 83.6 0 6.5 4.6 14.4 17.3 12.1C428.2 457.8 496 362.9 496 252 496 113.3 383.5 8 244.8 8zM97.2 352.9c-1.3 1-1 3.3.7 5.2 1.6 1.6 3.9 2.3 5.2 1 1.3-1 1-3.3-.7-5.2-1.6-1.6-3.9-2.3-5.2-1zm-10.8-8.1c-.7 1.3.3 2.9 2.3 3.9 1.6 1 3.6.7 4.3-.7.7-1.3-.3-2.9-2.3-3.9-2-.6-3.6-.3-4.3.7zm32.4 35.6c-1.6 1.3-1 4.3 1.3 6.2 2.3 2.3 5.2 2.6 6.5 1 1.3-1.3.7-4.3-1.3-6.2-2.2-2.3-5.2-2.6-6.5-1zm-11.4-14.7c-1.6 1-1.6 3.6 0 5.9 1.6 2.3 4.3 3.3 5.6 2.3 1.6-1.3 1.6-3.9 0-6.2-1.4-2.3-4-3.3-5.6-2z" />
</svg>
</a>
<div class="h-[2px] w-[200px] bg-gray-200"></div>
<a
role="button"
data-twe-ripple-init
data-twe-ripple-color="light"
class="inline-block rounded-full bg-primary px-6 pb-2 pt-2.5 text-xs font-medium uppercase leading-normal text-white shadow-[0_4px_9px_-4px_#3b71ca] transition duration-150 ease-in-out hover:bg-primary-600 hover:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.3),0_4px_18px_0_rgba(59,113,202,0.2)] focus:bg-primary-600 focus:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.3),0_4px_18px_0_rgba(59,113,202,0.2)] focus:outline-none focus:ring-0 active:bg-primary-700 active:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.3),0_4px_18px_0_rgba(59,113,202,0.2)] dark:shadow-[0_4px_9px_-4px_rgba(59,113,202,0.5)] dark:hover:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.2),0_4px_18px_0_rgba(59,113,202,0.1)] dark:focus:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.2),0_4px_18px_0_rgba(59,113,202,0.1)] dark:active:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.2),0_4px_18px_0_rgba(59,113,202,0.1)]">
See my projects
<svg
xmlns="http://www.w3.org/2000/svg"
fill="none"
viewBox="0 0 24 24"
stroke-width="1.5"
stroke="currentColor"
class="ms-1 inline h-5 w-5">
<path
stroke-linecap="round"
stroke-linejoin="round"
d="M19.5 8.25l-7.5 7.5-7.5-7.5" />
</svg>
</a>
</div>
</div>
<!-- Outer wrapper for flexbox -->
</div>
<!-- First column -->
<!-- Second column -->
<div class="">
<!-- Carousel -->
<div
id="carouselExampleCaptions"
class="relative"
data-twe-carousel-init
data-twe-ride="carousel">
<!--Carousel indicators-->
<div
class="absolute bottom-0 left-0 right-0 z-[2] mx-[15%] mb-4 flex list-none justify-center p-0"
data-twe-carousel-indicators>
<!-- Indicator 1 -->
<button
type="button"
data-twe-target="#carouselExampleCaptions"
data-twe-slide-to="0"
data-twe-carousel-active
class="mx-[3px] box-content h-2 w-2 flex-initial cursor-pointer border-0 border-y-[10px] border-solid border-transparent bg-white bg-clip-padding p-0 -indent-[999px] opacity-50 transition-opacity duration-[600ms] ease-[cubic-bezier(0.25,0.1,0.25,1.0)] motion-reduce:transition-none"
aria-current="true"
aria-label="Slide 1"></button>
<!-- Indicator 2 -->
<button
type="button"
data-twe-target="#carouselExampleCaptions"
data-twe-slide-to="1"
class="mx-[3px] box-content h-2 w-2 flex-initial cursor-pointer border-0 border-y-[10px] border-solid border-transparent bg-white bg-clip-padding p-0 -indent-[999px] opacity-50 transition-opacity duration-[600ms] ease-[cubic-bezier(0.25,0.1,0.25,1.0)] motion-reduce:transition-none"
aria-label="Slide 2"></button>
<!-- Indicator 3 -->
<button
type="button"
data-twe-target="#carouselExampleCaptions"
data-twe-slide-to="2"
class="mx-[3px] box-content h-2 w-2 flex-initial cursor-pointer border-0 border-y-[10px] border-solid border-transparent bg-white bg-clip-padding p-0 -indent-[999px] opacity-50 transition-opacity duration-[600ms] ease-[cubic-bezier(0.25,0.1,0.25,1.0)] motion-reduce:transition-none"
aria-label="Slide 3"></button>
</div>
<!--Carousel items-->
<div
class="relative w-full overflow-hidden rounded-bl-[4rem] shadow-2xl after:clear-both after:block after:content-['']">
<!--First item-->
<div
class="relative float-left -mr-[100%] w-full transition-transform duration-[600ms] ease-in-out motion-reduce:transition-none"
data-twe-carousel-active
data-twe-carousel-item
style="backface-visibility: hidden">
<!-- Image -->
<img
src="https://mdbootstrap.com/img/new/textures/full/243.jpg"
class="block h-screen w-full object-cover object-left"
alt="15 years of experience in the IT industry" />
<div
class="absolute inset-x-[15%] bottom-5 hidden py-5 text-center text-white md:block">
<h5 class="mb-3 text-xl">
15 years of experience in the IT industry
</h5>
<p class="mb-3">
I am in love with technology and have spent half my
life developing it
</p>
</div>
</div>
<!--Second item-->
<div
class="relative float-left -mr-[100%] hidden w-full transition-transform duration-[600ms] ease-in-out motion-reduce:transition-none"
data-twe-carousel-item
style="backface-visibility: hidden">
<!-- Image -->
<img
src="https://mdbootstrap.com/img/new/textures/full/102.jpg"
class="block h-screen w-full object-cover object-left"
alt="243 completed projects" />
<div
class="absolute inset-x-[15%] bottom-5 hidden py-5 text-center text-white md:block">
<h5 class="mb-3 text-xl">243 completed projects</h5>
<p class="mb-3">
I love challenges and treat each project as my own
</p>
</div>
</div>
<!--Third item-->
<div
class="relative float-left -mr-[100%] hidden w-full transition-transform duration-[600ms] ease-in-out motion-reduce:transition-none"
data-twe-carousel-item
style="backface-visibility: hidden">
<!-- Image -->
<img
src="https://mdbootstrap.com/img/new/textures/full/107.jpg"
class="block h-screen w-full object-cover object-left"
alt="53 satisfied customers" />
<div
class="absolute inset-x-[15%] bottom-5 hidden py-5 text-center text-white md:block">
<h5 class="mb-3 text-xl">53 satisfied customers</h5>
<p class="mb-3">
There is no better reward for me than a happy customer
</p>
</div>
</div>
</div>
<!--Carousel controls - prev item-->
<button
class="absolute bottom-0 left-0 top-0 z-[1] flex w-[15%] items-center justify-center border-0 bg-none p-0 text-center text-white opacity-50 transition-opacity duration-150 ease-[cubic-bezier(0.25,0.1,0.25,1.0)] hover:text-white hover:no-underline hover:opacity-90 hover:outline-none focus:text-white focus:no-underline focus:opacity-90 focus:outline-none motion-reduce:transition-none"
type="button"
data-twe-target="#carouselExampleCaptions"
data-twe-slide="prev">
<span class="inline-block h-8 w-8">
<svg
xmlns="https://mdbootstrap.com/img/new/textures/full/107.jpg"
fill="none"
viewBox="0 0 24 24"
stroke-width="1.5"
stroke="currentColor"
class="h-6 w-6">
<path
stroke-linecap="round"
stroke-linejoin="round"
d="M15.75 19.5L8.25 12l7.5-7.5" />
</svg>
</span>
<span
class="!absolute !-m-px !h-px !w-px !overflow-hidden !whitespace-nowrap !border-0 !p-0 ![clip:rect(0,0,0,0)]"
>Previous</span
>
</button>
<!--Carousel controls - next item-->
<button
class="absolute bottom-0 right-0 top-0 z-[1] flex w-[15%] items-center justify-center border-0 bg-none p-0 text-center text-white opacity-50 transition-opacity duration-150 ease-[cubic-bezier(0.25,0.1,0.25,1.0)] hover:text-white hover:no-underline hover:opacity-90 hover:outline-none focus:text-white focus:no-underline focus:opacity-90 focus:outline-none motion-reduce:transition-none"
type="button"
data-twe-target="#carouselExampleCaptions"
data-twe-slide="next">
<span class="inline-block h-8 w-8">
<svg
xmlns="http://www.w3.org/2000/svg"
fill="none"
viewBox="0 0 24 24"
stroke-width="1.5"
stroke="currentColor"
class="h-6 w-6">
<path
stroke-linecap="round"
stroke-linejoin="round"
d="M8.25 4.5l7.5 7.5-7.5 7.5" />
</svg>
</span>
<span
class="!absolute !-m-px !h-px !w-px !overflow-hidden !whitespace-nowrap !border-0 !p-0 ![clip:rect(0,0,0,0)]"
>Next</span
>
</button>
</div>
<!-- Carousel -->
</div>
<!-- Second column -->
</div>
<!-- Grid -->
</section>
<!-- Section: Split screen -->
</header>
<!--Main Navigation-->
<!--Main layout-->
<main class="h-[500px]"></main>
<!--Main layout-->
<!--Footer-->
<footer></footer>
<!--Footer-->
<!-- Main js file -->
<script type="module" src="src/js/index.js"></script>
<!-- Custom scripts -->
<script type="text/javascript"></script>
</body>
</html>
And this is how our src/js/index.js
file should look like:
// Initialization for ES Users
import { Carousel, initTWE } from 'tw-elements';
initTWE({ Carousel });
// Animated navbar
// We store the navbar element in a variable using the getElementById method and the id of the navbar
const animatedNavbar = document.getElementById('animated-navbar');
// We store the navbar-icons 'div' in a variable using the getElementById method and the id of the 'div' containing icons
const navbarIcons = document.getElementById('navbar-icons');
// We store the 'a' elements in a variable using the querySelectorAll method and the 'a' elements inside the navbar-icons 'div'
const navIconLinks = navbarIcons.querySelectorAll('a');
// If windows.scrollY > 0 (that means the user has scrolled down) we add an event listener
window.addEventListener('scroll', function () {
if (window.scrollY > 0) {
// We add the .bg-white class to the navbar when user scrolls down
animatedNavbar.classList.add('bg-white');
// We remove the .lg:py-4 class and add the .lg:py-5 class to the navbar when user scrolls down
animatedNavbar.classList.remove("lg:py-4");
animatedNavbar.classList.add("lg:py-5");
// We remove the .text-white class from the 'a' elements inside the navbar-icons 'div' and add the .text-neutral-500 class
navIconLinks.forEach((link) => {
link.classList.remove('text-white');
link.classList.add('text-neutral-500');
});
} else {
// We remove the .bg-white class from the navbar when user scrolls up to the top of the page
animatedNavbar.classList.remove('bg-white');
// We remove the .lg:py-5 class and add the .lg:py-4 class to the navbar when user scrolls up to the top of the page
animatedNavbar.classList.remove("lg:py-5");
animatedNavbar.classList.add("lg:py-4");
// We add the .text-white class to the 'a' elements inside the navbar-icons 'div' and remove the .text-neutral-500 class when user scrolls up to the top of the page
navIconLinks.forEach((link) => {
link.classList.add('text-white');
link.classList.remove('text-neutral-500');
});
}
});
If something is unclear or doesn't work as it should - hit me up on Twitter 😉
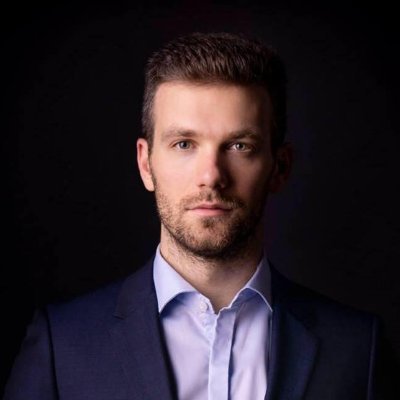
About author
Michal Szymanski
Co Founder at TW Elements and MDBootstrap / Listed in Forbes „30 under 30" / Open-source enthusiast / Dancer, nerd & book lover.
Author of hundreds of articles on programming, business, marketing and productivity. In the past, an educator working with troubled youth in orphanages and correctional facilities.