This guide describes in detail how to use the individual modules of the TW elements package to improve project performance and reduce the size of imported files.
CSS optimization
Tailwind is optimized to create as little CSS as possible, so there is no
need to do anything else other than configure
tailwind.config.js
file.
You can also use PurgeCSS as it is great tool that analyzes content of your html/javascript files and reduces CSS by deleting unused selectors.
If you are using PostCSS
plugin, another possibility to further
optimize your app is to add cssnano
at the end of the
postcss.config.js
npm install -D cssnano
module.exports = {
plugins: {
tailwindcss: {},
autoprefixer: {},
...(process.env.NODE_ENV === 'production' ? { cssnano: {} } : {})
}
}
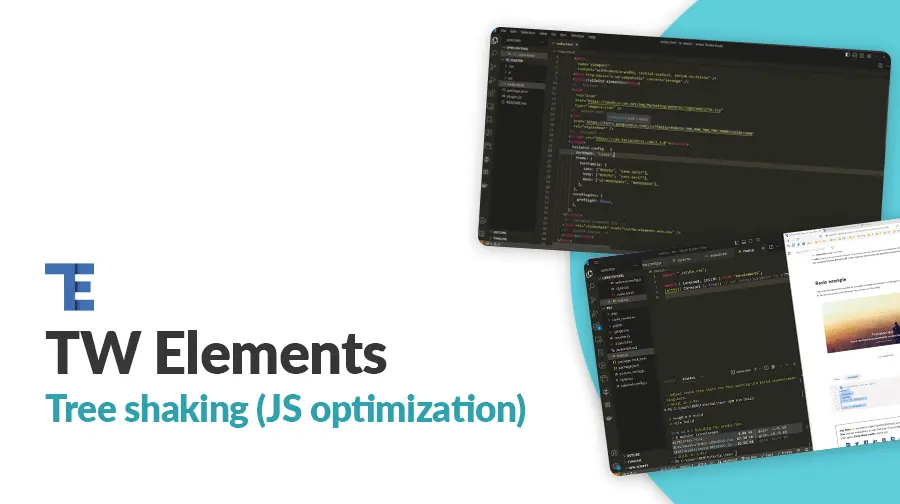
JS optimization
TW elements provides a ES format that allows, instead of importing the entire library, to choose only the modules that are used in the project. Thanks to this, we significantly reduce the amount of KB downloaded by the application. It can be reduced even several times!
For Example: In a new vite application, importing and
using only input
component, the build weights close to
30 kB
. Using the same code but importing the entire library,
the build weights a little bit over 300 kB
.
The only thing you have to remember is to always init the component using
initTWE
method provided in tw-elements
library
otherwise the components may not work properly.
How to use the ES format of the TW elements?
Here's a short instruction for importing a single module on the example of
a
Select
component:
Step 1
Create a new project with TW elements. If you need step by step tutorial on how to create a new vite app and connect it with TW Elements, go to the Getting Started Vite page
mdb init tailwind-elements
Step 2
Install dependencies
npm install
Step 3
Add code of the basic example.
<div class="flex justify-center">
<div class="mb-3 xl:w-96">
<select data-twe-select-init>
<option value="1">One</option>
<option value="2">Two</option>
<option value="3">Three</option>
<option value="4">Four</option>
<option value="5">Five</option>
<option value="6">Six</option>
<option value="7">Seven</option>
<option value="8">Eight</option>
</select>
</div>
</div>
Step 4
Import Select
from modules instead of importing only
tw-elements
.
initTWE
method unless you are using the
UMD
format.
import { Select, initTWE } from "tw-elements";
initTWE({ Select }, options);
The options
are documented in the
TW Elements
formats.
Step 5
Run the application
npm start
Step 6 *optional
Run the build and compare the created JS weight. You can play around with other components and compare the builds.
npm run build
Tooling support
VS Code + Tailwind CSS IntelliSense
TW elements is registered as a plugin inside
tailwind.config.js
. That allows for our custom css classes to
be picked up by the Tailwind CSS IntelliSense. If the IntelliSense is not
showing our classes check whether the content
inside
tailwind.config.js
is defined properly. We encourage to use it
as it makes the live of a developer much easier.