Simple card
Use the following simple card component with a title and description.
Loading...
import React from 'react';
import { TERipple } from 'tw-elements-react';
export default function CardBasicExample(): JSX.Element {
return (
<div
className="block rounded-lg bg-white p-6 shadow-[0_2px_15px_-3px_rgba(0,0,0,0.07),0_10px_20px_-2px_rgba(0,0,0,0.04)] dark:bg-neutral-700">
<h5
className="mb-2 text-xl font-medium leading-tight text-neutral-800 dark:text-neutral-50">
Card title
</h5>
<p className="mb-4 text-base text-neutral-600 dark:text-neutral-200">
Some quick example text to build on the card title and make up the
bulk of the card's content.
</p>
<TERipple>
<button
type="button"
className="inline-block rounded bg-primary px-6 pb-2 pt-2.5 text-xs font-medium uppercase leading-normal text-white shadow-[0_4px_9px_-4px_#3b71ca] transition duration-150 ease-in-out hover:bg-primary-600 hover:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.3),0_4px_18px_0_rgba(59,113,202,0.2)] focus:bg-primary-600 focus:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.3),0_4px_18px_0_rgba(59,113,202,0.2)] focus:outline-none focus:ring-0 active:bg-primary-700 active:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.3),0_4px_18px_0_rgba(59,113,202,0.2)] dark:shadow-[0_4px_9px_-4px_rgba(59,113,202,0.5)] dark:hover:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.2),0_4px_18px_0_rgba(59,113,202,0.1)] dark:focus:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.2),0_4px_18px_0_rgba(59,113,202,0.1)] dark:active:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.2),0_4px_18px_0_rgba(59,113,202,0.1)]">
Button
</button>
</TERipple>
</div>
);
}
Card with image
You can use the following example of a card element with an image for blog posts, user cards, and many more.
Loading...
import React from 'react';
import { TERipple } from 'tw-elements-react';
export default function CardWithImageExample(): JSX.Element {
return (
<div
className="block rounded-lg bg-white shadow-[0_2px_15px_-3px_rgba(0,0,0,0.07),0_10px_20px_-2px_rgba(0,0,0,0.04)] dark:bg-neutral-700">
<a href="#!">
<img
className="rounded-t-lg"
src="https://tecdn.b-cdn.net/img/new/standard/nature/184.jpg"
alt="" />
</a>
<div className="p-6">
<h5
className="mb-2 text-xl font-medium leading-tight text-neutral-800 dark:text-neutral-50">
Card title
</h5>
<p className="mb-4 text-base text-neutral-600 dark:text-neutral-200">
Some quick example text to build on the card title and make up the
bulk of the card's content.
</p>
<TERipple>
<button
type="button"
className="inline-block rounded bg-primary px-6 pb-2 pt-2.5 text-xs font-medium uppercase leading-normal text-white shadow-[0_4px_9px_-4px_#3b71ca] transition duration-150 ease-in-out hover:bg-primary-600 hover:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.3),0_4px_18px_0_rgba(59,113,202,0.2)] focus:bg-primary-600 focus:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.3),0_4px_18px_0_rgba(59,113,202,0.2)] focus:outline-none focus:ring-0 active:bg-primary-700 active:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.3),0_4px_18px_0_rgba(59,113,202,0.2)] dark:shadow-[0_4px_9px_-4px_rgba(59,113,202,0.5)] dark:hover:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.2),0_4px_18px_0_rgba(59,113,202,0.1)] dark:focus:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.2),0_4px_18px_0_rgba(59,113,202,0.1)] dark:active:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.2),0_4px_18px_0_rgba(59,113,202,0.1)]">
Button
</button>
</TERipple>
</div>
</div>
);
}
With ripple effect
Indicate the point of touch with user input to screen reactions using ripple on card image.
Loading...
import React from 'react';
import { TERipple } from 'tw-elements-react';
export default function CardWithRippleExample(): JSX.Element {
return (
<div
className="block rounded-lg bg-white shadow-[0_2px_15px_-3px_rgba(0,0,0,0.07),0_10px_20px_-2px_rgba(0,0,0,0.04)] dark:bg-neutral-700">
<TERipple>
<div
className="relative overflow-hidden bg-cover bg-no-repeat">
<img
className="rounded-t-lg"
src="https://tecdn.b-cdn.net/img/new/standard/nature/186.jpg"
alt="" />
<a href="#!">
<div
className="absolute bottom-0 left-0 right-0 top-0 h-full w-full overflow-hidden bg-[hsla(0,0%,98%,0.15)] bg-fixed opacity-0 transition duration-300 ease-in-out hover:opacity-100"></div>
</a>
</div>
</TERipple>
<div className="p-6">
<h5
className="mb-2 text-xl font-medium leading-tight text-neutral-800 dark:text-neutral-50">
Card title
</h5>
<p className="mb-4 text-base text-neutral-600 dark:text-neutral-200">
Some quick example text to build on the card title and make up the
bulk of the card's content.
</p>
<TERipple>
<button
type="button"
className="inline-block rounded bg-primary px-6 pb-2 pt-2.5 text-xs font-medium uppercase leading-normal text-white shadow-[0_4px_9px_-4px_#3b71ca] transition duration-150 ease-in-out hover:bg-primary-600 hover:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.3),0_4px_18px_0_rgba(59,113,202,0.2)] focus:bg-primary-600 focus:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.3),0_4px_18px_0_rgba(59,113,202,0.2)] focus:outline-none focus:ring-0 active:bg-primary-700 active:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.3),0_4px_18px_0_rgba(59,113,202,0.2)] dark:shadow-[0_4px_9px_-4px_rgba(59,113,202,0.5)] dark:hover:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.2),0_4px_18px_0_rgba(59,113,202,0.1)] dark:focus:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.2),0_4px_18px_0_rgba(59,113,202,0.1)] dark:active:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.2),0_4px_18px_0_rgba(59,113,202,0.1)]">
Button
</button>
</TERipple>
</div>
</div>
);
}
Card with header and footer
Add optional header and footer sections to the card.
Loading...
import React from 'react';
import { TERipple } from 'tw-elements-react';
export default function CardWithHeaderAndFooterExample(): JSX.Element {
return (
<div
className="block rounded-lg bg-white text-center shadow-[0_2px_15px_-3px_rgba(0,0,0,0.07),0_10px_20px_-2px_rgba(0,0,0,0.04)] dark:bg-neutral-700">
<div
className="border-b-2 border-neutral-100 px-6 py-3 dark:border-neutral-600 dark:text-neutral-50">
Featured
</div>
<div className="p-6">
<h5
className="mb-2 text-xl font-medium leading-tight text-neutral-800 dark:text-neutral-50">
Special title treatment
</h5>
<p className="mb-4 text-base text-neutral-600 dark:text-neutral-200">
With supporting text below as a natural lead-in to additional
content.
</p>
<TERipple>
<button
type="button"
className="inline-block rounded bg-primary px-6 pb-2 pt-2.5 text-xs font-medium uppercase leading-normal text-white shadow-[0_4px_9px_-4px_#3b71ca] transition duration-150 ease-in-out hover:bg-primary-600 hover:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.3),0_4px_18px_0_rgba(59,113,202,0.2)] focus:bg-primary-600 focus:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.3),0_4px_18px_0_rgba(59,113,202,0.2)] focus:outline-none focus:ring-0 active:bg-primary-700 active:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.3),0_4px_18px_0_rgba(59,113,202,0.2)] dark:shadow-[0_4px_9px_-4px_rgba(59,113,202,0.5)] dark:hover:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.2),0_4px_18px_0_rgba(59,113,202,0.1)] dark:focus:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.2),0_4px_18px_0_rgba(59,113,202,0.1)] dark:active:shadow-[0_8px_9px_-4px_rgba(59,113,202,0.2),0_4px_18px_0_rgba(59,113,202,0.1)]">
Button
</button>
</TERipple>
</div>
<div
className="border-t-2 border-neutral-100 px-6 py-3 dark:border-neutral-600 dark:text-neutral-50">
2 days ago
</div>
</div>
);
}
Horizontal
Use card which has its child elements aligned horizontally.
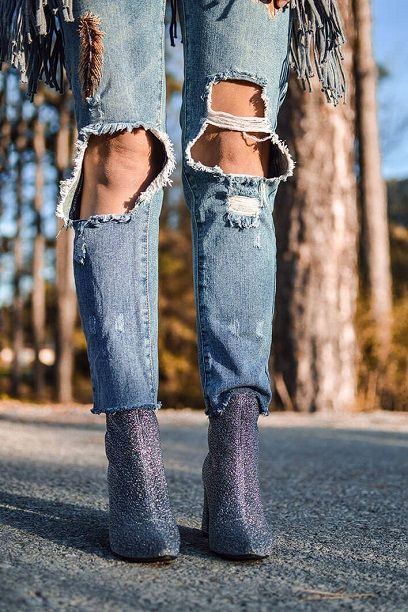
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
Last updated 3 mins ago
import React from 'react';
export default function App() {
return (
<div
className="flex flex-col rounded-lg bg-white shadow-[0_2px_15px_-3px_rgba(0,0,0,0.07),0_10px_20px_-2px_rgba(0,0,0,0.04)] dark:bg-neutral-700 md:max-w-xl md:flex-row">
<img
className="h-96 w-full rounded-t-lg object-cover md:h-auto md:w-48 md:rounded-none md:rounded-l-lg"
src="https://tecdn.b-cdn.net/wp-content/uploads/2020/06/vertical.jpg"
alt="" />
<div className="flex flex-col justify-start p-6">
<h5
className="mb-2 text-xl font-medium text-neutral-800 dark:text-neutral-50">
Card title
</h5>
<p className="mb-4 text-base text-neutral-600 dark:text-neutral-200">
This is a wider card with supporting text below as a natural lead-in
to additional content. This content is a little bit longer.
</p>
<p className="text-xs text-neutral-500 dark:text-neutral-300">
Last updated 3 mins ago
</p>
</div>
</div>
);
}
Related resources
If you are looking for more advanced options, try Bootstrap Cards from MDBootstrap.